Welcome to my Portfolio! I am Zhuang Xinjie, a computer science undergraduate at NUS.
Here you can find details on my contributions to the Software Engineering project.
PROJECT: MeetingBook
MeetingBook is a desktop scheduler application targeted at university students involved in multiple Co-Curricular Activities and Project-Based modules. The application is a modified contact book with features to group contacts and assign meetings to such groups. MeetingBook is written by a team of 5 students from National University of Singapore’s School of Computing. It is forked from AddressBook-Level4 written by SE-EDU.
Summary of contributions
-
Major enhancement 1 : added the
Group
class-
What it does: allow categorisation of persons according to their project group, discussion group and CCA etc.
-
Justification: This feature allows user to categorise his/her contacts based on their group associations.
-
Highlights: This feature is in parallel with person. While it does not affect existing functions, interactions between the two in the future can be complex.
-
-
Major enhancement 2 : added the ability to add and delete a
Group
into and from the MeetingBook.-
What it does: allow creation and deletion of customised
Group
in the MeetingBook. -
Justification: This feature allows user to modify the existing categories of people.
-
Highlights: This feature makes use of
UniqueGroupList
in the MeetingBook to manipulate the list of groups.
-
-
Major enhancement 3 : added the ability to join and remove an existing contact person into an existing group in the MeetingBook.
-
What it does: allow to establish membership relation between persons and groups in MeetingBook.
-
Justification: This feature allows user to modify how existing persons are categorised.
-
Highlights: This feature makes use of
UniqueGroupList
in person, andUniquePersonList
in group to keep track of membership relation among them.
-
-
Code contributed: [Pull requests][Reposense]
-
Other contributions:
Contributions to the User Guide
Shown below are my contributions to the User Guide. They demonstrate my ability to write documentation and manuals targeting end-users. |
Adding a new group: addGroup
Add a new group with user input title into MeetingBook.
Format: addGroup n/[Name]
Examples:
-
addGroup n/CS2103T
Adds a new group with title 'CS2103T' into the MeetingBook. -
addGroup n/Discussion Group 1
Adds a new group with title 'Discussion Group 1' into the MeetingBook.
Deleting a group: deleteGroup
Remove the group that matches use input title.
Format: deleteGroup n/[Name]
Examples:
-
deleteGroup n/CS2103T
Removes the group with title 'CS2103T', which is added previously, from MeetingBook. -
deleteGroup n/Discussion Group 1
Removes the group with title 'Discussion Group 1', which is added previously, from MeetingBook.
Joining a person to group: join
Add a person, specified by name, into a group, specified by the group title.
Format: join n/[Name] g/[Group]
Example:
-
join n/Derek g/CS2103T
Makes the person 'Derek' to be a member of group 'CS2103T'.
Both the person and group should exist in the MeetingBook. |
Removing a person from group: leave
Remove a person, specified by name, from a group, specified by the group title.
Format: leave n/[Name] g/[Group]
Example:
-
leave n/Derek g/CS2101
Removes the person 'Derek' from the group 'CS2101'.
Both the person and group should exist in the MeetingBook. The person should also be an existing member of the group. |
Contributions to the Developer Guide
Shown below are my contributions to the Developer Guide. They demonstrate my ability to write technical documentation and highlight the depth and technical details of my contributions to the software engineering project MeetingBook. |
Model component
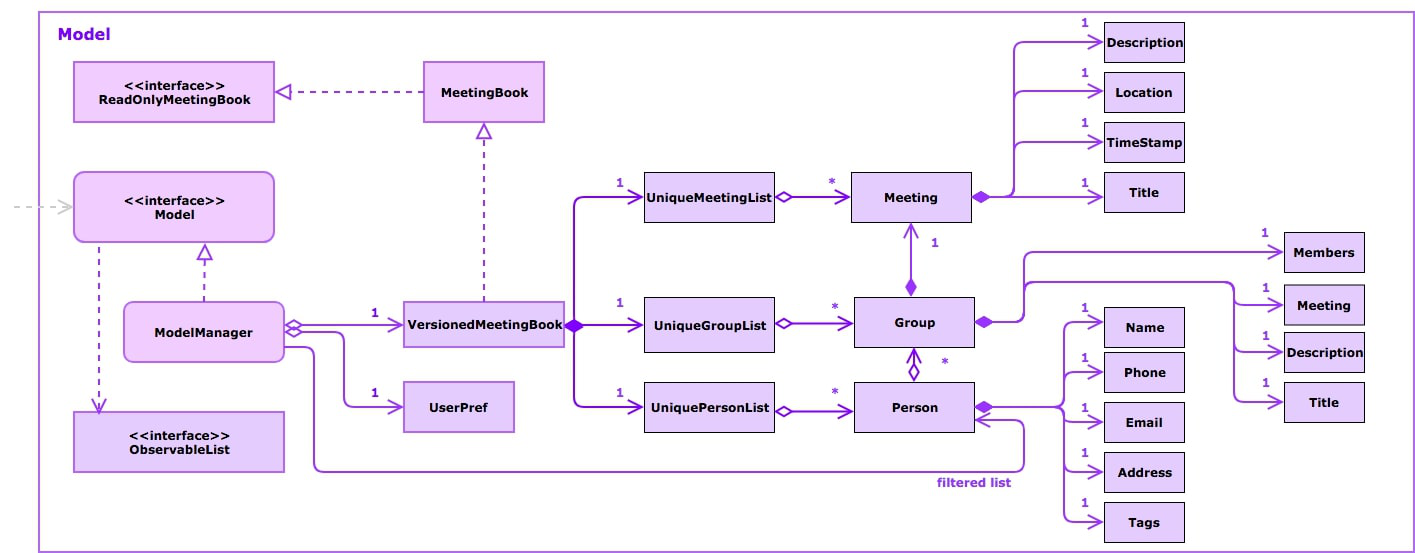
API : Model.java
As a more OOP model, we can store a Tag list and a Group list in MeetingBook , which Person can reference.
This would allow MeetingBook to only require one Tag object per unique Tag , and one Group object per
unique Group , instead of each Person needing their own Tag and Group object.
|
Group feature
Current Implementation
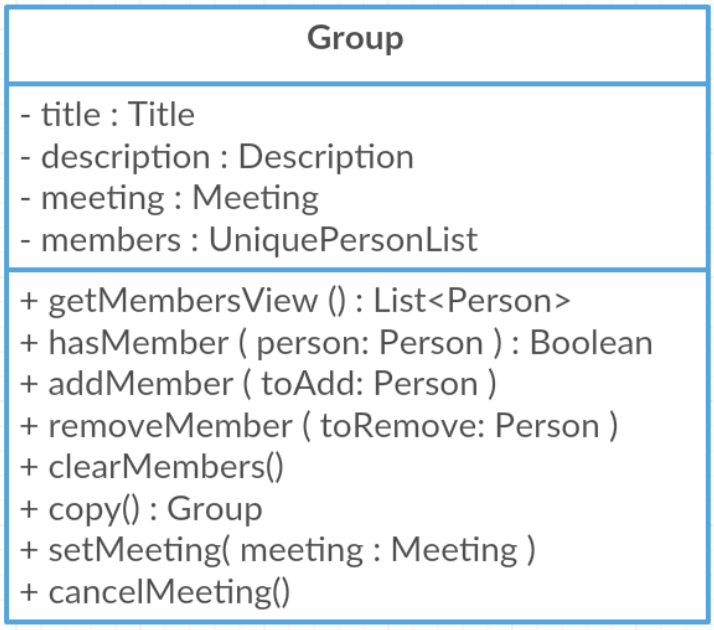
API : Group.java
The Group
,
-
is identified by
Title
andDescription
. -
contains the
Meeting
details for this particular group. This is an optional field. -
keeps track of its members in a
UniquePersonList
. The methodhasMember(Person person)
can check the enrollment of a particularPerson
. -
supports add and remove member using methods
addMember(Person toAdd)
andremoveMember(Person toRemove)
. -
exposes an unmodifiable
List<Person>
for observation of member enrollment status bygetMembersView()
. -
supports set and cancel of
Meeting
of this group by methodssetMeeting(Meeting meeting)
andcancelMeeting()
.
Usage of Group class
A Group
can be added and removed from MeetingBook
using addGroup
and deleteGroup
command respectively.
Once the Group
exists in the MeetingBook
, the existing Person
can be assigned to that group using join
command.
The person can also be removed from the group by leave
command.
Each Group
can keep track of a list of its members. This is supported by UniquePersonList
class.
Each Person
can also keep a list of groups he/she enrols in. An UniqueGroupList
is added to support this functionality.
Design Consideration
Aspect: Management of group relationship
-
Alternative 1 (current choice): The
add
andremove
operations support bidirectional update of relationships.-
Pros: Less complexity in current project structure.
-
Cons: The future optimisation of these operations is less flexible.
-
-
Alternative 2: Use a central
groupManager
to manage the group-person relationships on theMeetingBook level
.-
Pros: Greater room for possible future improvement, and ability to contain more complex relationships required by potential users.
-
Cons: The idea is less compatible with the current project structure. Extensive change in structure and logic must be performed, and is error-prone.
-
UniqueGroupList feature
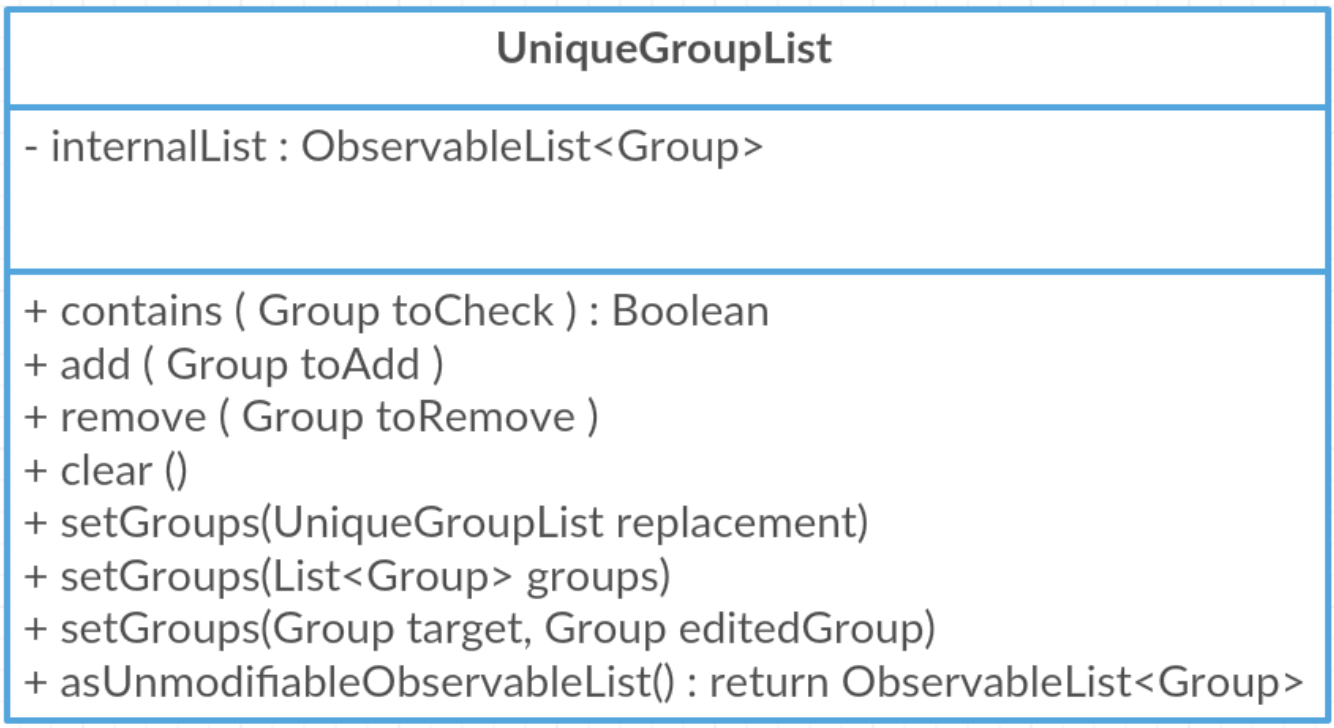
API : UniqueGroupList.java
The UniqueGroupList
,
-
ensures a list of
Group
objects without duplicates. -
supports
add
,remove
andreplace
operations on groups in the list. -
exposes an unmodifiable
ObservableList<Group>
for observation of the list.
Both UniqueGroupList and UniquePersonList implements Iterable interface.
|
Person feature
Current Implementation
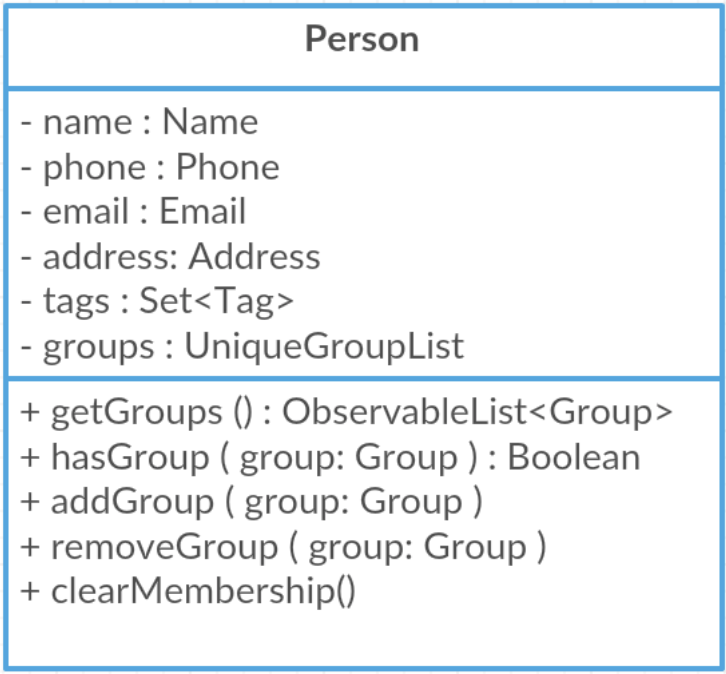
API : Person.java
-
Person
now supports group identification. Every person will keep a list of groups that he/she has enrolled in. -
Every
Person
in MeetingBook can be added or removed from an existing group. Use the methodsaddGroup()
andremoveGroup
. -
Person
can check whether he/she is in a particular group byhasGroup(Group group)
method.
AddGroup and DeleteGroup feature
The AddGroup and DeleteGroup commands modify the UniqueGroupList
in the versionedMeetingBook
.
This section shows how the addGroup and deleteGroup commands are implemented.
addGroup
command usage
The addGroup
command allows user to add a new group with user input title to the MeetingBook.
This command is executed with the following syntax:
Syntax: addGroup n/[Name]
Example: addGroup n/CS2103T
: adds a new group with title 'CS2103T' into the MeetingBook.
The follow sequence diagram shows how addGroup command functions.
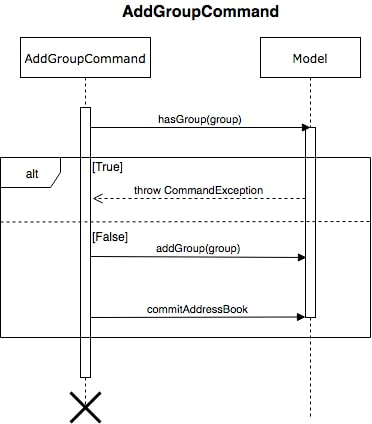
deleteGroup
command usage
The deleteGroup
command allows user to remove an existing group from the MeetingBook.
This command is executed with the following syntax:
Syntax: deleteGroup n/[Name]
Example: deleteGroup n/CS2101
: removes the existing group with title 'CS2101' from the MeetingBook.
The follow sequence diagram shows how deleteGroup command functions.
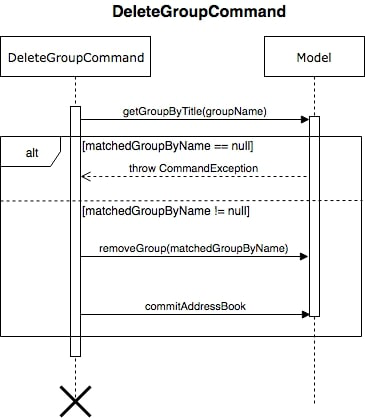
Implementation of addGroup
and deleteGroup
commands
The versionedMeetingBook
maintains a UniqueGroupList
to keep track of all groups
that exist in this MeetingBook. The commands thus modify and update this list of groups through ModelManager
.
Join and Leave feature
The Join and Leave commands modify the relationship between groups existed in the UniqueGroupList
and
people in the UniquePersonList
maintained by `versionedMeetingBook. This section provides description of the usage
and implementation of these commands.
join
command usage
The join
command updates relationship between a person and a group existed in the MeetingBook.
The person specified by name now becomes a member of the group specified by title.
This command is executed with the following syntax:
Syntax: join n/[Name] g/[Group]
Example: join n/Derek g/CS2101
: makes the person 'Derek' become a member of group 'CS2101'
The follow sequence diagram shows how join command functions.
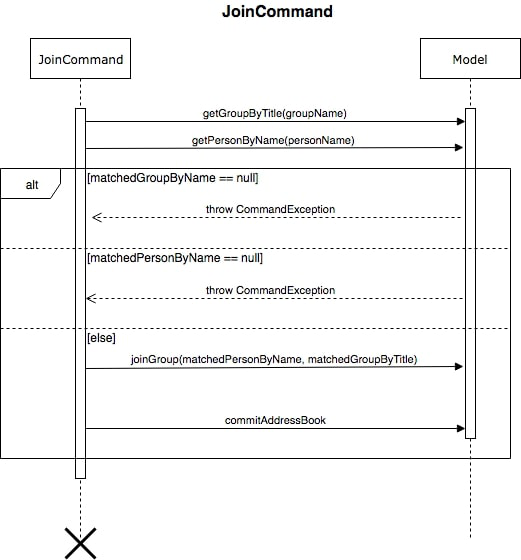
leave
command usage
The leave
command updates relationship between person and group in the same way as the join
command.
The person specified by name now stops to be a member of the group specified by title.
And the person is removed from this group. This command is executed with the following syntax:
Syntax: leave n/[Name] g/[Group]
Example: leave n/Ben g/CS2103T
: removes the person Ben
from the group CS2103T
.
The follow sequence diagram shows how leave command functions.
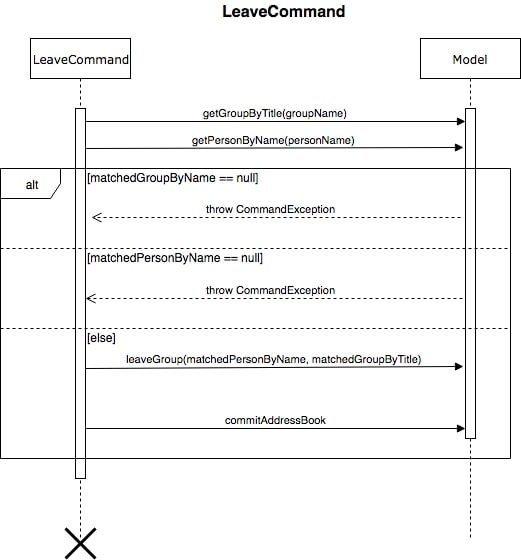
Implementation of join
and leave
commands
The versionedMeetingBook
maintains a UniqueGroupList
to keep track of all groups that exist in this MeetingBook.
It also maintains a UniquePersonList
to keep track of all people that exist in this MeetingBook.
The commands thus modify and update a pair of person and group, as specified by user input, in their respective list
through ModelManager
.