Introduction
Welcome to my project portfolio! I am Ooi Heng Seng, a year 3 Computer Science student in National University of Singapore.
This document showcases the contributions I made to Software Engineering’s project MeetingBook.
Project: MeetingBook
Overview
We are a team of 5 students from National University of Singapore’s School of Computing. We developed a desktop scheduling application called MeetingBook for our software engineering’s project. The application is created for scheduling meetings for NUS modules and CCAs. The user interacts with it using a command line interface (CLI), and has a graphical user interface (GUI) created with JavaFX. We have to deliver the product within 9 weeks. The project is based on a software engineering project AddressBook-Level4. The project is written in Java, and has about 10kLoC.
Summary of contributions
-
Major enhancement: updated the user interface
-
How it looks like:
-
What it does: allows the user to view all information at a glance.
-
Justification: the updated user interface improves the user experience significantly because it reduces the amount of interaction to look for the information.
-
Highlights: this enhancement requires additional enhancement to select, find and list commands to interact with all interface elements. It requires user to enter additional parameter to commands mentioned.
-
Credits: JavaFX
-
-
Major enhancement: updated the select command
-
Updated select command to select between groups, meetings or persons. Select groups will filter meeting and person lists to show only relevant information of the group.
-
-
Minor enhancement:
-
Updated list command to show all groups, meetings or persons.
-
-
Code contributed: [RepoSense][Pull Requests]
-
Other contributions:
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users.
User Interface
This section will explain the user interface of the application.
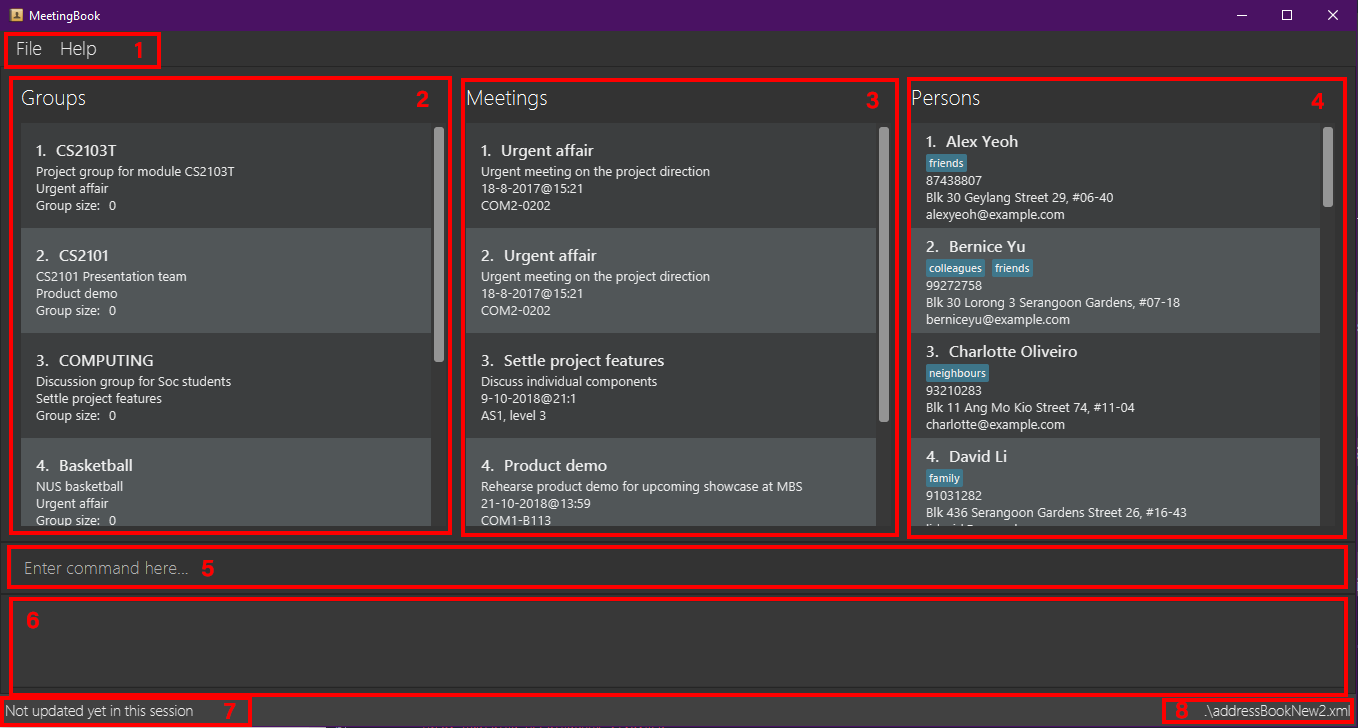
-
Menu bar: provides access to functions
-
Group list: displays a list of groups
-
Meeting list: displays a list of meetings
-
Person list: displays a list of person
-
Command box: receives command from user and execute
-
Result display: displays the result of command executed
-
Update status: displays the update status of current session
-
Save path: displays current data save path
Selecting a group / person / meeting: select
Format: select [person|group|meeting] [index]
where [index]
is a positive integer (starts from 1)
Examples:
-
select g/1
: selects the first group in the filtered group list. -
select m/3
: selects the third meeting in the filtered meeting list. -
select p/2
: selects the second person in the filtered person list.
Listing all groups / meetings / persons : list
Display all existing groups on the group list / meetings on the meeting list / persons on the person list display.
Format: list <group|meeting|person|all>
Shorthand: list <g|m|p|a>
If the list is unfiltered, the list will remain the same. If list command does not have parameters entered, it will execute list all by default. |
Examples:
-
list group
: list all groups. -
list m
: list all meetings. -
list person
: list all persons. -
list a
: list all groups, meetings and persons.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project.
UI component
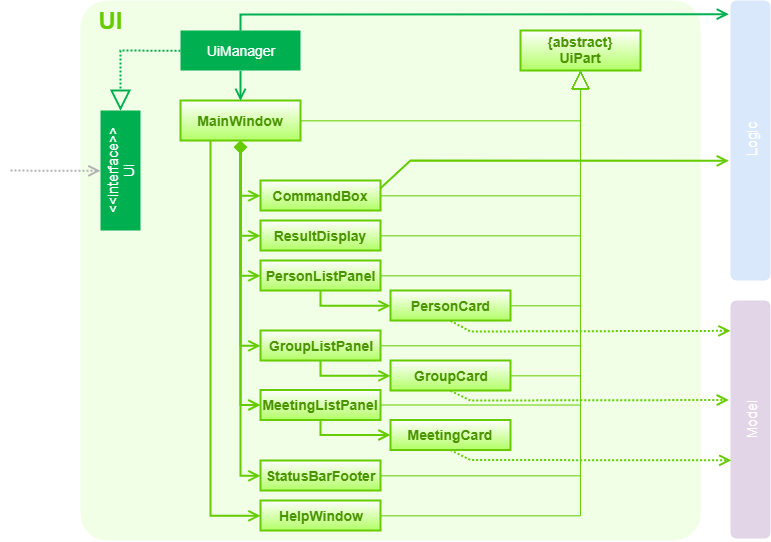
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
, GroupListPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
Select feature
The select command selects the GroupCard
, MeetingCard
or PersonCard
in the ObservableList<Group>
, ObservableList<Meeting>
,
or ObservableList<Person>
respectively in Model
that is bounded to the UI. This section will explain current implementation
of select command, execution sequence, and design considerations of multiple implementations.
Current Implementation
The select mechanism is facilitated by ModelManager
.
It extends Model
with three lists, UniquePersonList
, UniqueMeetingList
and UniqueGroupList
and implements the following operations:
-
Model#updateFilteredPersonList
— Updates person list with a specificPredicate
. -
Model#updateFilteredGroupList
— Updates group list with specificPredicate
. -
Model#updateFilteredMeetingList
— Updates meeting list with specificPredicate
.
Given below is an example usage scenario and how the select mechanism behaves at each step.
Step 1. The user launches the application for the first time.
The ModelManager
will be initialized with the initial MeetingBook state, and UniquePersonList
, UniqueMeetingList
and UniqueGroupList
contains all person, meetings and groups respectively without filter.
Step 2. The user executes select
command to select the item type with the specified index in the MeetingBook.
The SelectCommandParser
parses the command to determine the select type, and the command executes the following steps:
-
Step 2.1. If the prefix is
g/
, aJumpToGroupListRequestEvent
is sent to update the UI to select the group card,Model#updateFilteredPersonList
is called to filterUniquePersonList
to contain only person that are associated with the group, andModel#updateFilteredMeetingList
is called to filterUniqueMeetingList
to show only meeting that is associated with the group. -
Step 2.2. If the prefix is
m/
, aJumpToMeetingListRequestEvent
is sent to the UI to select the meeting card. -
Step 2.3. If the prefix is
p/
, aJumpToListRequestEvent
is sent to the UI to select the person card.
If a group is selected, subsequent select calls to person and meeting list will only select the person or meeting in the filtered
UniquePersonList or UniqueMeetingList respectively.
|
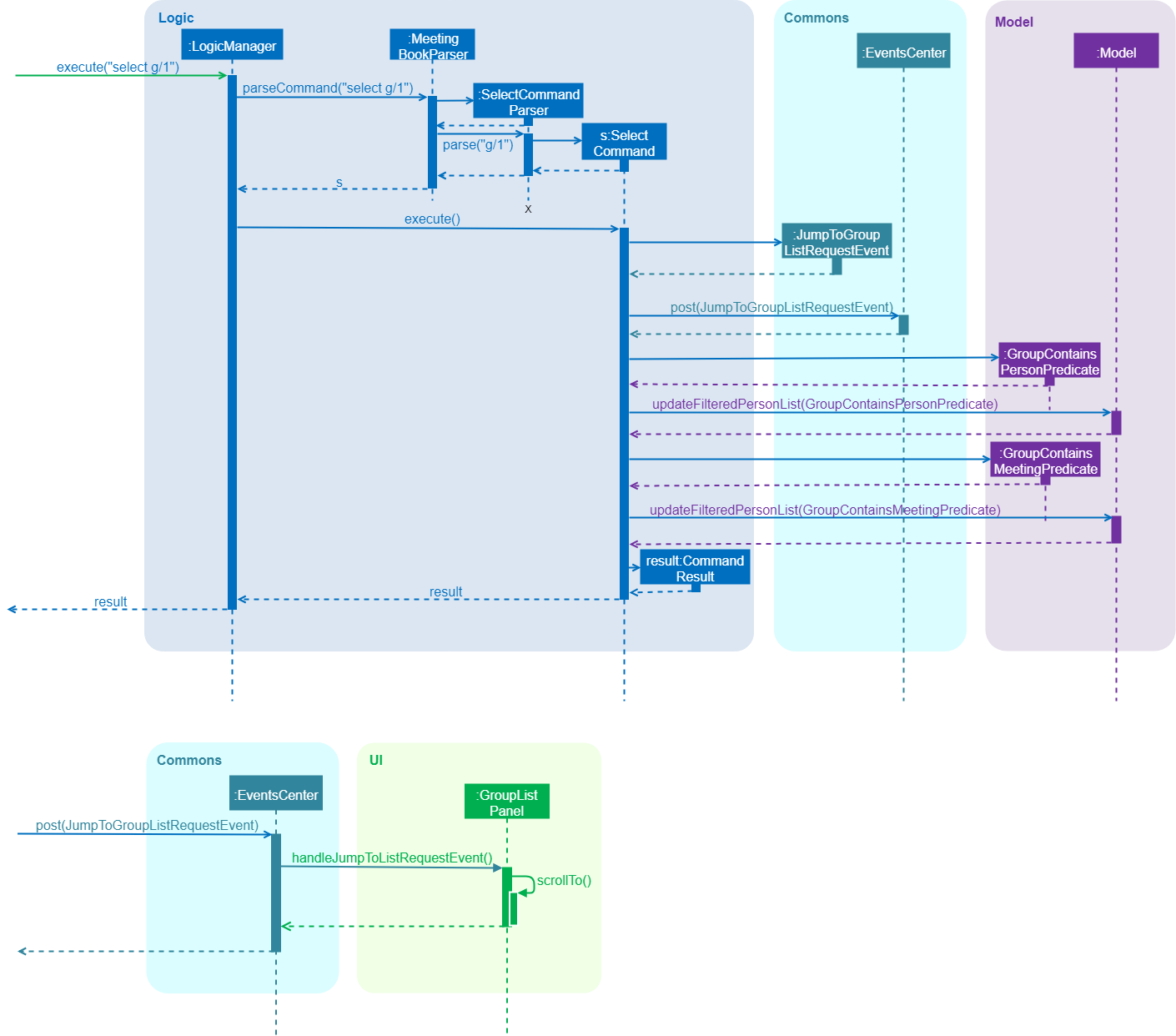
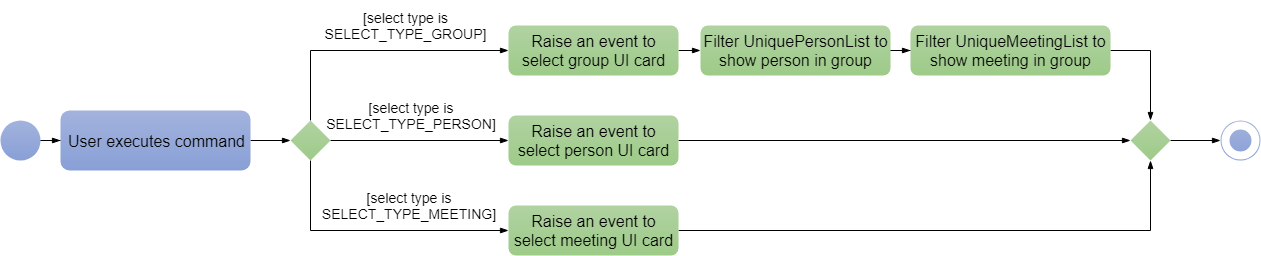
Design Considerations
Aspect: Data structure to support the select command
-
Alternative 1 (current choice): Use a single select class to perform all select operations.
-
Pros: Easy to implement as there are fewer lines of code required.
-
Cons: Harder to extend if need to implement select command for other items, select class will become bloated if too many items require select command.
-
-
Alternative 2: Abstract select class containing required variables and methods without implementation, and select commands inherit from the abstract class and implement the execution functionality.
-
Pros: Good data structure, easy to extend functionality to select other items.
-
Cons: Requires more code to implement.
-
List feature
The list
command removes any filters applied to UniqueGroupList
, UniquePersonList
and UniqueMeetingList
by other
commands. This section will explain current implementation of list command and design considerations during development
phase.
Current implementation
The list mechanism is facilitated by ModelManager
. It uses the same operations as mentioned in select
feature,
namely Model#updateFilteredPersonList
, Model#updateFilteredGroupList
and Model#updateFilteredMeetingList
.
Given below is an example usage scenario and operations executed by list mechanism at each step.
Step 1. The user launches the application. ModelManager
will be initialized with initial MeetingBook state, and
UniquePersonList
, UniqueMeetingList
and UniqueGroupList
contains all person, meetings and groups respectively
without filter.
Step 2. The user executes list
command with additional parameter to specify the list to show all items. Available
parameters are person
, meeting
, group
and all
, or their shorthand equivalent p
, m
, g
and a
.
ListCommandParser
parses the command to determine the list type, and the command executes the following steps:
-
Step 2.1. If the parameter is
group
/g
,Model#updateFilteredGroupList
is called withPREDICATE_SHOW_ALL_GROUPS
to remove any filters applied toUniqueGroupList
. -
Step 2.2. If the parameter is
meeting
/m
,Model#updateFilteredMeetingList
is called withPREDICATE_SHOW_ALL_MEETINGS
to remove any filters applied toUniqueMeetingList
. -
Step 2.3. If the parameter is
person
/p
,Model#updateFilteredPersonList
is called withPREDICATE_SHOW_ALL_PERSONS
to remove any filters applied toUniquePersonList
. -
Step 2.4. If the parameter is
all
/a
/ empty, steps 2.1., 2.2. and 2.3. will be executed.
List will remain unchanged if it does not have any filters applied before executing list command.
|
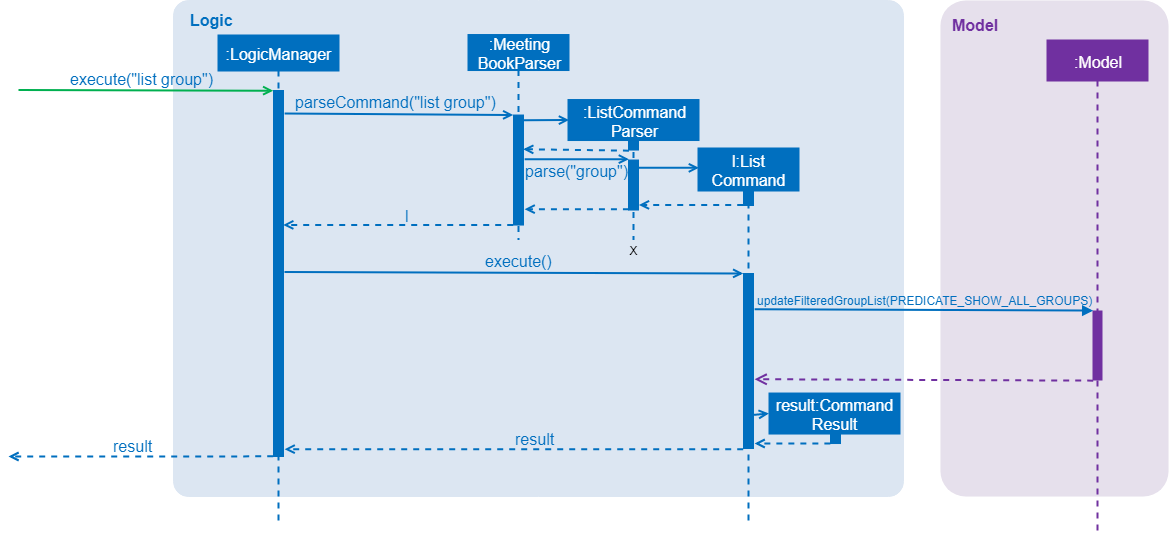
Below is the pseudo code of list
command execution.
public CommandResult execute(Model model, CommandHistory history) {
requireNonNull(model);
switch (listCommandType) {
case PERSON:
// Show all persons, return success
case GROUP:
// Show all groups, return success
case MEETING:
// Show all meetings, return success
default:
// Show all persons, groups and meetings, return success
}
}
Design considerations
Aspect: Data structure to support the list command
-
Alternative 1 (current choice) : Use a single list class to perform all list operations.
-
Pros: Easy to implement as fewer lines of code are required.
-
Cons: Harder to extend list functionality if need to implement list command for other items, list class will become bloated if too many items require list command.
-
-
Alternative 2 : Abstract list class containing required variables and methods without implementation, and specific list commands inherit from the abstract class and implement the execution functionality.
-
Pros: Good data structure, easy to extend functionality to other items that require list command.
-
Cons: Requires more lines of code to implement.
-