Welcome to Pakorn Ueareeworakul’s Portfolio!
Here you can find details on my contributions to various Software Engineering projects. Specifically, this portfolio contains links to functional and test code I wrote, and excerpts from technical documentation I created.
PROJECT: MeetingBook
1. Overview
MeetingBook is a desktop scheduler and contact book hybrid designed for university students involved in multiple Co-Curricular Activities and Project-Based modules. By using our application, you can group your contacts according to their project groups, and create reminders on upcoming meetings with the groups you created, so that you don’t miss your meeting again.
MeetingBook is forked from AddressBook-Level4 written by SE-EDU by our team which composed of 5 students from National University of Singapore’s School of Computing.
2. Summary of contributions
-
Major enhancement: added the ability to schedule/cancel meetings
-
What it does: allows the user to add/remove meetings from the specified group.
-
Justification: This feature is essential to morph the AddressBook into a meeting scheduler.
-
Highlights: This enhancement requires major modifications to the Model and addition of new classes such as Meeting and TimeStamp. The implementation is also challenging as it requires extensive coordination between team members due to the level of dependency it has on the group feature.
-
-
Code contributed: [code]
-
Other contributions:
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
3. Contributions to the User Guide
Shown below are my contributions to the User Guide. They demonstrates my ability to write documentation and manuals targeting end-users. |
3.1. Schedule meetings: meet
To schedule a meeting, use the meet
command.
Format: meet GROUP_NAME n/MEETING_NAME t/MEETING_TIME l/MEETING_LOCATION d/MEETING_DESCRIPTION
-
Schedules a meeting with the group
GROUP_NAME
-
Either all or none of the fields must be provided.
-
In case that none of the fields is provided, the command will cancel the meeting associated with the group.
-
Scheduling a new meeting to the same group overwrites the old meeting.
MEETING_TIME is specified in the format dd-mm-yyyy@hh:mm
|
Examples:
-
Example 1:
-
meet Presentation2101 n/Demo Rehearsal t/26-10-2018@12:30 l/COM1-0218 d/Meeting for Project Demo
(This schedules the meeting)Figure 1. Result of the command, note the meeting 'Demo Rehearsal'.
-
-
Example 2:
-
meet Presentation2101 n/Demo Rehearsal t/26-10-2018@12:30 l/COM1-0218 d/Meeting for Project Demo
(This schedules the meeting)Figure 2. Result of the command, note the meeting 'Demo Rehearsal'. -
meet Presentation2101 n/Emergency Meeting t/26-10-2018@12:30 l/COM1-0218 d/Some slides are wrong
(This overwritesDemo Rehearsal
withEmergency Meeting
)Figure 3. Result of the command, note the 'Emergency Meeting' now replaces 'Demo Rehearsal'.
-
-
Example 3:
-
meet OtherGroup n/Emergency Meeting t/26-10-2018@12:30 l/COM1-0218 d/Some slides are wrong
(This fails becauseOtherGroup
is not in the MeetingBook)Figure 4. Result of the command, note the error message'.
-
It is assumed that the group Presentation2101 is present and is the only group in the MeetingBook and has no meeting as of the moment before each example starts.
|
3.2. Cancel meetings: cancel
To cancel a meeting, use the cancel
command.
Format: cancel GROUP_NAME
Example:
-
cancel Project2103
(This cancels the meeting withProject2103
) -
cancel Project2103
cancel Project2103
(This fails because the meeting is already cancelled)
It is assumed that the group Project2103 is present in the MeetingBook and has a meeting.
|
calling meet GROUP_NAME without argument is equivalent to calling cancel GROUP_NAME .
|
4. Contributions to the Developer Guide
Shown below are my contributions to the Developer Guide. They demonstrates my ability to write technical documentation and highlights the depth and technical details of my contributions to the software engineering project MeetingBook. |
4.1. Meeting Feature
Meeting
is one of the central feature of this application. It allows the user to create a reminder of an upcoming event associated with a Group
.
4.1.1. Current implementation
Meetings are encapsulated using the Meeting
class.
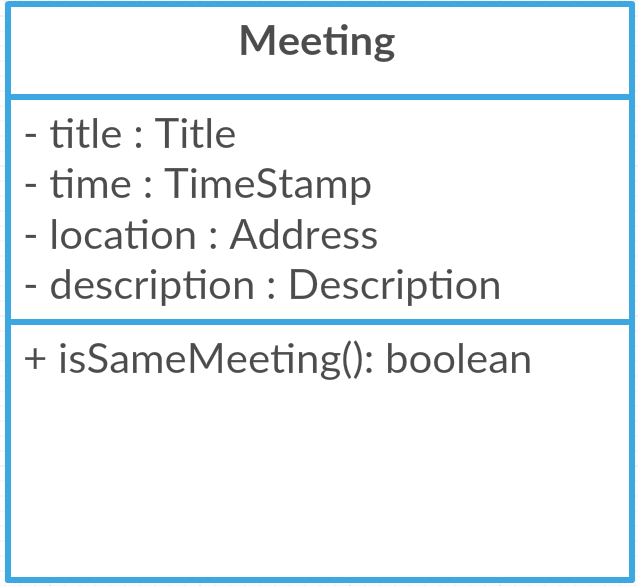
API : Meeting.java
Meeting
have the following properties:
-
It contains the title, time, location and description of the meeting it describes.
-
Each
Group
object can have a maximum of one meeting. -
Two
Meetings
are considered similar if they share the same title, time, and location.
4.1.2. Using the Meeting class
In the current implementation, Meeting
is related to Group
by composition. To facilitate the meeting feature, Group
implements the following operations:
-
Group#hasMeeting()
— This returnstrue
if there is a meeting associated with the group -
Group#getMeeting()
— This group’s meeting ornull
if the group has no meeting. -
Group#setMeeting(Meeting meeting)
— This assignsmeeting
to the group. -
Group#cancelMeeting()
— This removes the meeting associated witht the group.
These operations can be accessed through the model using the following operations:
-
Model#setMeeting(Group group, Meeting meeting)
— This assigns the meetingmeeting
to the groupgroup
. -
Model#cancelMeeting(Group group)
— This cancels the meeting associated with the groupgroup
Given below is an example usage scenario and how the model behaves at each step.
Step 1. The application is launched.
We assumes that the groups Project2103 , Presentation and FrisbeeTeam are present in the MeetingBook, and that none of the groups mentioned has a meeting scheduled.
|
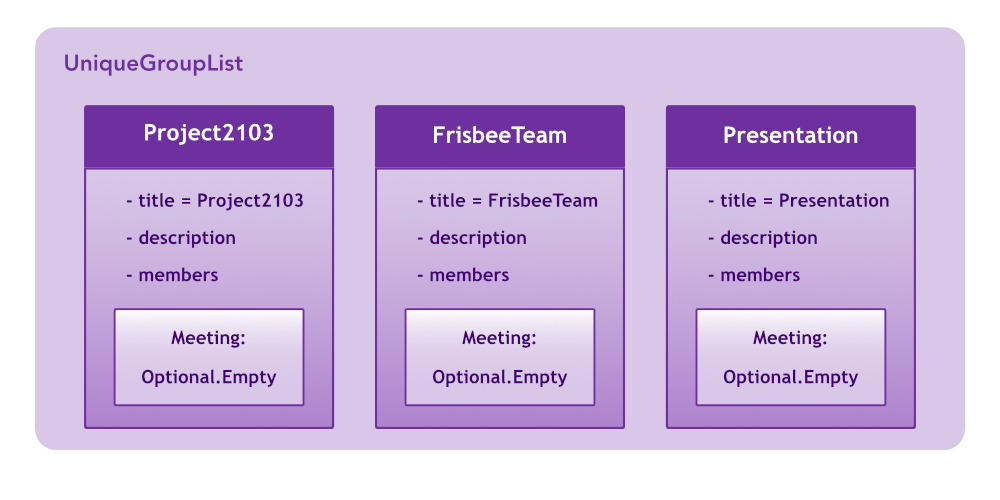
Meeting field in a group without a meeting is an empty Optional
|
Step 2. The command meet FrisbeeTeam n/Sunday Practice t/28-10-2018@10:00 l/UTown Green d/Practice for Inter-College Games
is executed. This schedules a meeting named Sunday Practice
with the group FrisbeeTeam
.
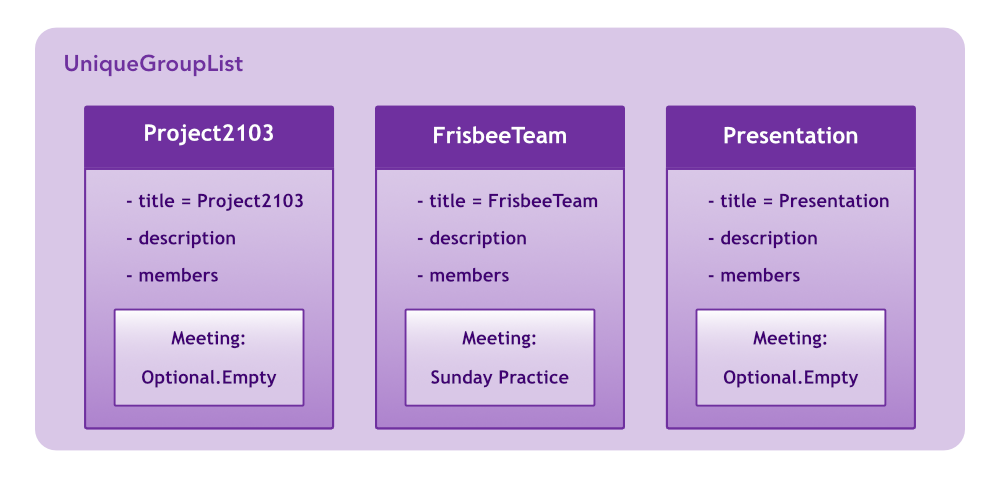
Step 3. The command meet Project2103 n/Weekly Meeting…
is executed. This schedules a meeting named Weekly Meeting
with the group Project2103
.
…
represents any valid arguments that legally completes the command.
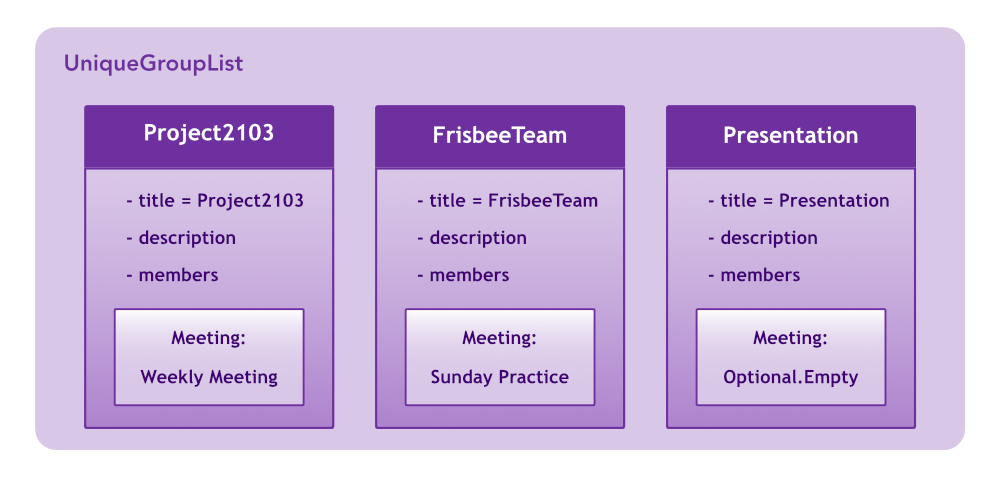
Step 4. The command cancel FrisbeeTeam
is executed. This removes the meeting Sunday Practice
from the group FrisbeeTeam
.
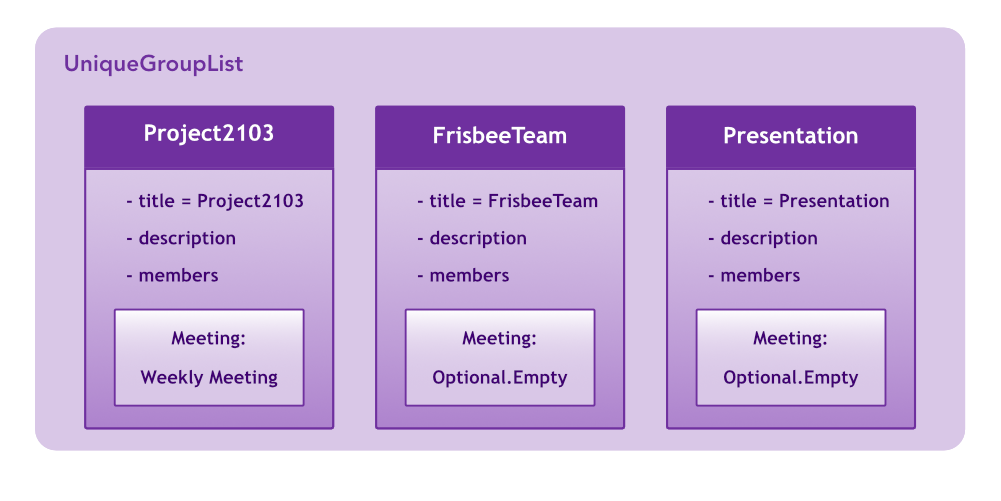
Calling cancel on a group without meeting returns an error
|
calling meet GROUP_NAME without any arguments is equivalent to calling cancel GROUP_NAME .
|
Step 5. The command meet Project2103 n/Demo Preparation…
is executed. This overwrites the meeting Weekly Meeting
associated with the group Project2103
with the new meeting named Demo Preparation
.
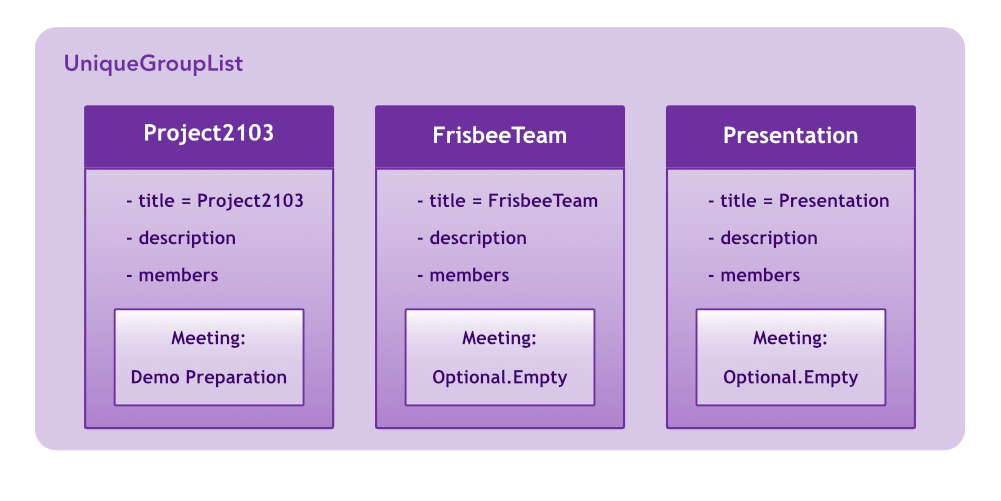
This operation creates a new Meeting object which replaces the old one.
|
The following sequence diagrams show how the meet and cancel command function.
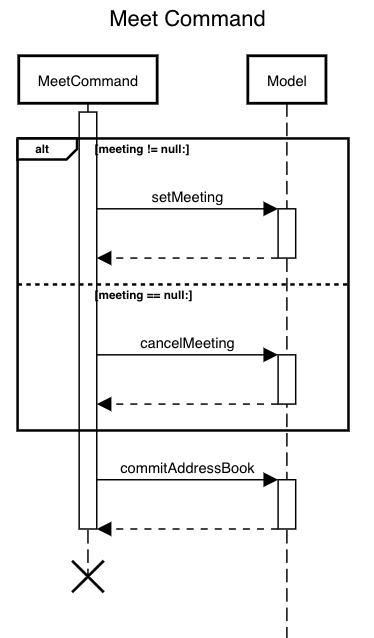
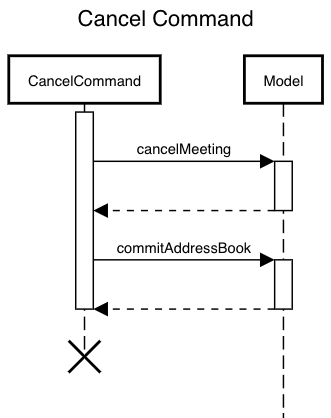
4.1.3. Design Considerations
Aspect: Storage of meetings
-
Alternative 1 (current choice): Storing meetings inside groups
-
Pros: This design simplifies the source code by not requiring a separate class to store meetings.
-
Cons: This design requires iterating through all groups to retreive the list of meetings
-
-
Alternative 2: Storing meetings in a separate uniqueMeetingList class
-
Pros: This design simplifies retreival of the list of meetings.
-
Cons: This design causes the code becomes more complicated and more difficult to test.
-
Aspect: Specification of target group for meet and cancel commands
-
Alternative 1 (current choice): Specifying the group using its name.
-
Pros: This design makes the command syntax natural and intuitive.
-
Cons: This design forces the user have to manually type in the group name.
-
-
Alternative 2: Specifying the group using its index.
-
Pros: This design is easier to use when the number of groups is high.
-
Cons: This design causes the command syntax to become unnatural, and makes the program more suspectible to errors.
-