Here you can find details on my contributions to various Software Engineering projects.
PROJECT: MeetingBook
MeetingBook is a desktop scheduler application developed in a course of 6 weeks under a module which aims to improve our proficiency in Software Engineering. MeetingBook is targeted at university students involved in multiple Co-Curricular Activities and Project-Based modules. MeetingBook is written by a team of 5 students from National University of Singapore’s School of Computing. We modified a contact book, forked from AddressBook-Level4 written by SE-EDU, to includes features such as grouping of contacts and assign meetings to such groups.
My contribution mainly includes the implementation of export and import features and project management.
1. Summary of contributions
-
Major enhancement: added the ability to export/import MeetingBook
-
What it does: allows the user to export/import XML version of MeetingBook.
-
Justification: allows the user to port over existing data of MeetingBook to other devices. This ensure that when the user change his device, he will not lose all information that stored in MeetingBook and is able to continue using MeetingBook without much disruption.
-
Highlights: requires clear understanding to the underlying mechanism on how the Storage component works and being used in MeetingBook. User can decide between different conflict resolution behaviors to resolve merging conflict occurs when importing MeetingBook.
-
-
Minor enhancement: Implementation of
filepath
command . This allow user of MeetingBook to view or change the storage location of MeetingBook. -
Code contributed: [code][Pull Requests]
-
Other contributions:
-
Project management:
-
Setting up project management tools such as Coveralls, Travis, Codacy for the team repository
-
Managed releases v1.1 - v1.3.1 (5 releases) on GitHub
-
Setting up of RepoSense configuration for the repository
-
-
Enhancements to existing features:
-
Refactoring of Address Book to MeetingBook: #157
-
-
Documentation:
-
Community:
-
PRs reviewed (with non-trivial review comments): #29
-
-
2. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
2.1. Exporting data : export
Export MeetingBook data to store as backup elsewhere or send the data to other users of MeetingBook.
A XML file containing MeetingBook data will be created at the filepath indicated by the user.
Format: export f/FILEPATH
Example:
-
export f/backup.xml
: Export MeetingBook asbackup.xml
.
The image below shows the result after successfully executing the command.
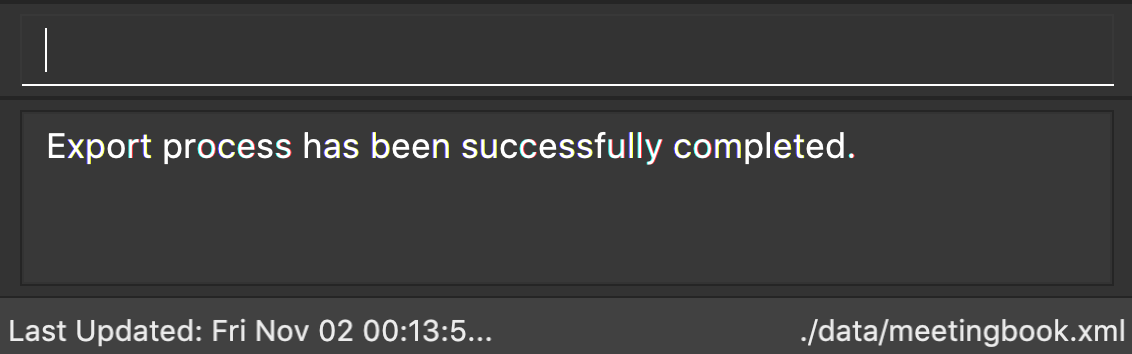
2.2. Importing data : import
Import other MeetingBook data and integrate into the current MeetingBook.
Default behaviour is to ignore any conflicting Person/Group entries unless --force
option is supplied.
Format: import [--force] f/FILEPATH
Example:
-
import f/backup.xml
: Import backup.xml into MeetingBook -
import --force f/backup.xml
: Import backup.xml into MeetingBook, overwriting all conflicting entries.
The image below shows the result after successfully executing the command.
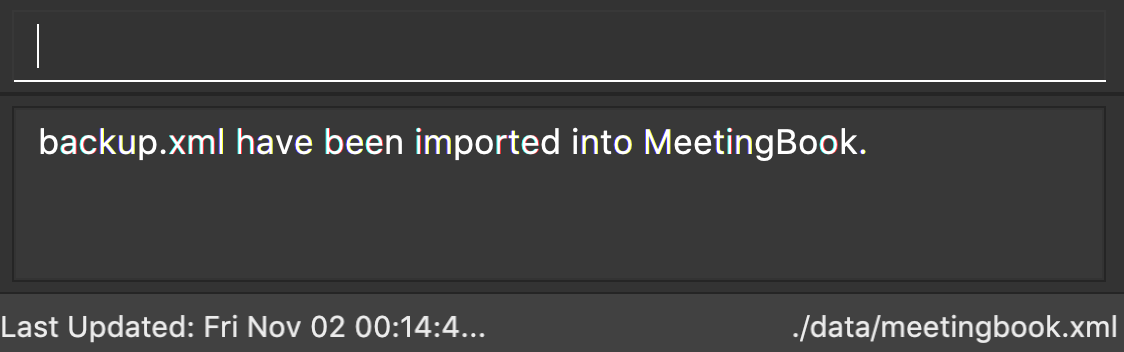
2.3. Changing Storage Location: filepath
Change the storage location of MeetingBook.
Format: filepath f/FILEPATH
Example:
-
filepath f/new_path.xml
: Storage location of MeetingBook is now stored atnew_path.xml
.
3. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
3.1. Export feature
3.1.1. Current Implementation
3.1.1.1. Overview
The export mechanism is facilitated by ExportCommand
. ExportCommandParser
is responsible for parsing the input arguments. The end result of this command is a Xtensible Markup Language (XML) file containing data of MeetingBook when is executed successfully.
If there is no filepath provided, export command will return an invalid message, which the usage information to the user.
|
The following sequence diagram shows how export operation works:
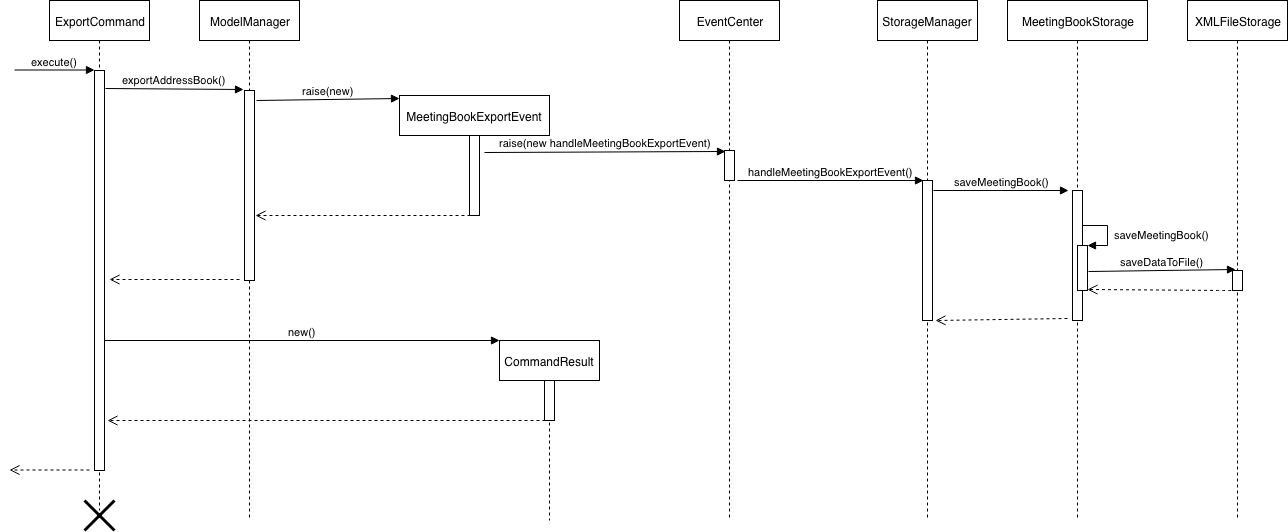
The program will raise a MeetingBookExportEvent
to EventCenter
. StorageManager
which subscribed to the EventCenter
will trigger StorageManager#handleMeetingBookExportEvent
when received MeetingBookExportEvent
.
This method will then attempt to export the data as a XML file using Model#exportMeetingBook
.
3.1.1.2. Export to a XML file
The translation from MeetingBook to XML formatted file is handled by XmlFileStorage#saveDataToFile
.
As MeetingBook stores Person
, Group
, Meeting
as Object
s, these information have to be properly formatted into Java Architecture for XML Binding (JAXB)-friendly version before it can be successfully saved.
Object
s are modelled using their respective XMLAdapted
classes which contain directions for it to parse into JAXB-friendly version. Marshal
Object will then marshal all the JAXB-friendly version of Person
, Meeting
, Group
and assemble it into a XML file.
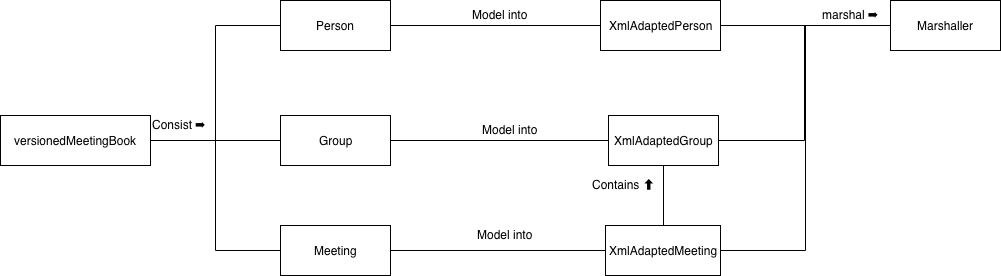
3.1.2. Design Consideration
3.1.2.1. Aspect: Make export undoable
-
Alternative 1 (current choice): Does not allow it to be undo.
-
Pros: Easy to implement and verify if the command is executed successfully.
-
Cons: Hard for the user as the user have to manually navigate through the directory to delete the XML file to achieve the same result as undoing the command.
-
-
Alternative 2: Allow it to be undoable.
-
Pros: Allow user to undo the command.
-
Cons: Make it difficult to implement as there is a need to consider on how to resolve the following scenarios:
-
After user export data, user may directly make changes to the XML file exported. Does undoing the operation deletes the file or not since it have been modified after the export command?
-
After user export data, user rename the XML file or move the XML file. This causes the original file referenced by the
export
command to be lost and unable to continue the undo operation.
-
These scenarios create unnecessary complications when we want to attempt undoing the operation. Therefore, we choose to not allow the command to be undoable.
-
3.2. Import feature
3.2.1. Current implementation
3.2.1.1. Overview
The import command allow the user to import a XML file containing of MeetingBook data into his MeetingBook.
This is facilitated by is facilitated by ImportCommand
. ImportCommandParser
is responsible for parsing the input arguments.
The end result of this command is entries of Person
, Group
, Meeting
added into the current MeetingBook.
3.2.1.2. Import from XML file
The translation from XML formatted file to MeetingBook
is handled by XmlFileStorage#loadDataToFile
.
As MeetingBook consists Person
, Group
, Meeting
as Object
s, these information have to be properly formatted back into Java Architecture for XML Binding (JAXB)-friendly version before it can then modelled into its respective classes (i.e. xmlAdaptedPerson
modelled back into Person
object).
Each Object
s has their respective XMLAdapted
class which contain directions to model back from JAXB-friendly version . Unmarshal
Object is solely responsible for disassemble the XML file into JAXB-friendly versions of Person
, Meeting
, Group
, which will then be feed as parameters to create another instance of MeetingBook
.
The imported MeetingBook
will then merge with the current MeetingBook
using MeetingBook#merge
.
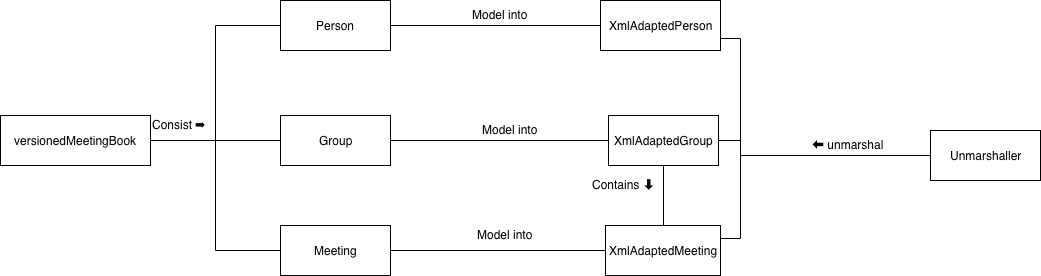
3.2.2. Handling of conflicting entries
The default behavior of handling conflicting entries is to ignore any conflicting entries. However, user can choose to change this behavior to overwrite any conflicting entries using --force
option when entering the command.
An example of the command set to the overwriting behaviour is import --force f/backup.xml
.
The code snippet below showcase how MeetingBook toggle between the behaviours.
public void merge(ReadOnlyMeetingBook imported, boolean overwrite) {
// If Both book contains same entries
if (equals(imported)) {
return;
}
if (!(imported instanceof MeetingBook)) {
return;
}
MeetingBook importedBook = (MeetingBook) imported;
importedBook.persons.iterator()
.forEachRemaining((person) -> mergePerson(person, overwrite));
importedBook.groups.iterator()
.forEachRemaining((group) -> mergeGroup(group, overwrite));
}
3.2.3. Handling of irrelevant imported data
The default conflicting resolving behavior (ignoring any conflict files) may cause Person
to lose their association with Group
.
Take this case for example. Current MeetingBook and the imported MeetingBook both have a Person named "Alex Lim" with the following attributes.
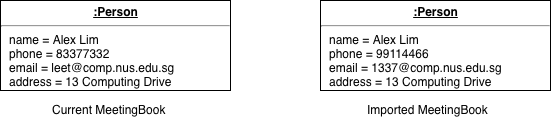
Person
Object in current MeetingBook and imported MeetingBookWhen the default conflicting resolving behaviour is used, "Alex Lim" of the imported MeetingBook will not be imported into current MeetingBook.
However, "Alex Lim" of the imported MeetingBook belongs to a Group
.
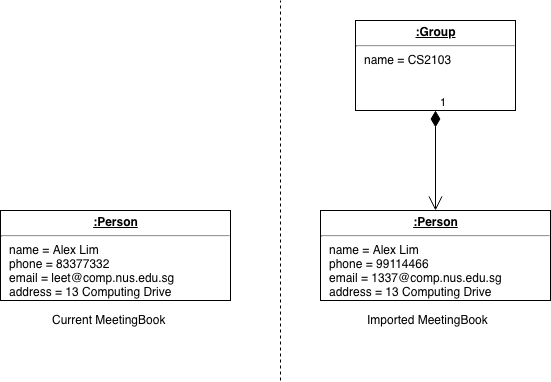
Person
of imported MeetingBook belonging to a Group
before merging to current MeetingBookTherefore, when importing the Group
that "Alex Lim" of the imported MeetingBook is in, the Group
will be imported without "Alex Lim" of the imported MeetingBook. The Group
will not contains "Alex Lim" of the current MeetingBook as these two "Alex Lim" are not of the same Person
. If "Alex Lim" of the imported MeetingBook is the sole member of the Group
, the Group
will still be created but with no members instead.
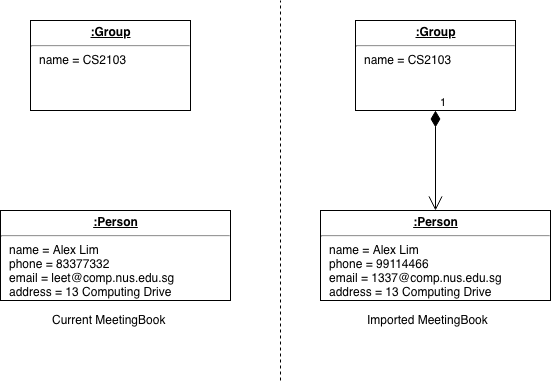
Person
of imported MeetingBook belonging to a Group
after merging to current MeetingBook3.2.4. Design Consideration
3.2.4.1. Aspect: Handling of conflict entries
-
Alternative 1 (current choice): Either ignore or overwrite conflicting entries.
-
Pros: Easy to handle conflicting cases.
-
Cons: Loss of data as information is destroyed or lost when importing. When we choose to ignore, we lose data from the XML file as they are not imported in. If we were to overwrite conflicting entries, we lose information in our current MeetingBook as they are overwritten by the imported data.
-
-
Alternative 2: Append an arbitrary number to the name of
Person
/Group
.-
Pros: No loss of data when importing.
-
Cons: End up with lots of data with arbitrary number appended to it. This may cause confusion to the user as there is many similar entries of the
Person
/Group
object only to be differ by an arbitrary number. This will also causefind
command to not work as expected.find
command currently matches exact search term and the arbitrary number appended cause it to no longer match the name of theObject
.
-